Well I would like your concepts on bit set and reset operations to be clear. No matter which language you use be it assembly or embedded c, you will always require to set and reset register bits.
Now whether you want to initialize some peripheral or poll something you will require these three operations.
Bit set operation
This means making a particular bit in an register as 1 or setting it. Now let us see this property of logical OR.
x OR 1 = 1
So whatever be the value of x if you OR it with 1 the result will be 1. This is what we want right? I’ll be taking one example after explaining bit reset.
Bit reset operation
This means that you want to make a particular bit of a register 0 or reset it. We make use of the property of logical AND:
x AND 0 = 0
So whatever be the value of x if you AND it with 0 the result is 0. This is what we wanted so bit reset is done by logical AND operation.
Masking
Often you are interested only in a particular bits of a register. This may be the input of some sensor or something else. Now you want to use masking in this scenario. So masking means you reset all the bits that are not required and let the bits under consideration as it is. Again we make use of logical AND properties.
x AND 0 = 0
x AND 1 = x
So you make the masking pattern by making the bits you want as 1 and the ones you don’t want as 0. Convert this value to hex and logical AND that mask value with the register.
Bit toggle
Bit toggle is also useful. So for this we make use of the property of ex-or as follows.
x ex-or 1 = complement of x or x-bar
Thus if you ex-or the bit with 1 the bit will be toggled.
Example
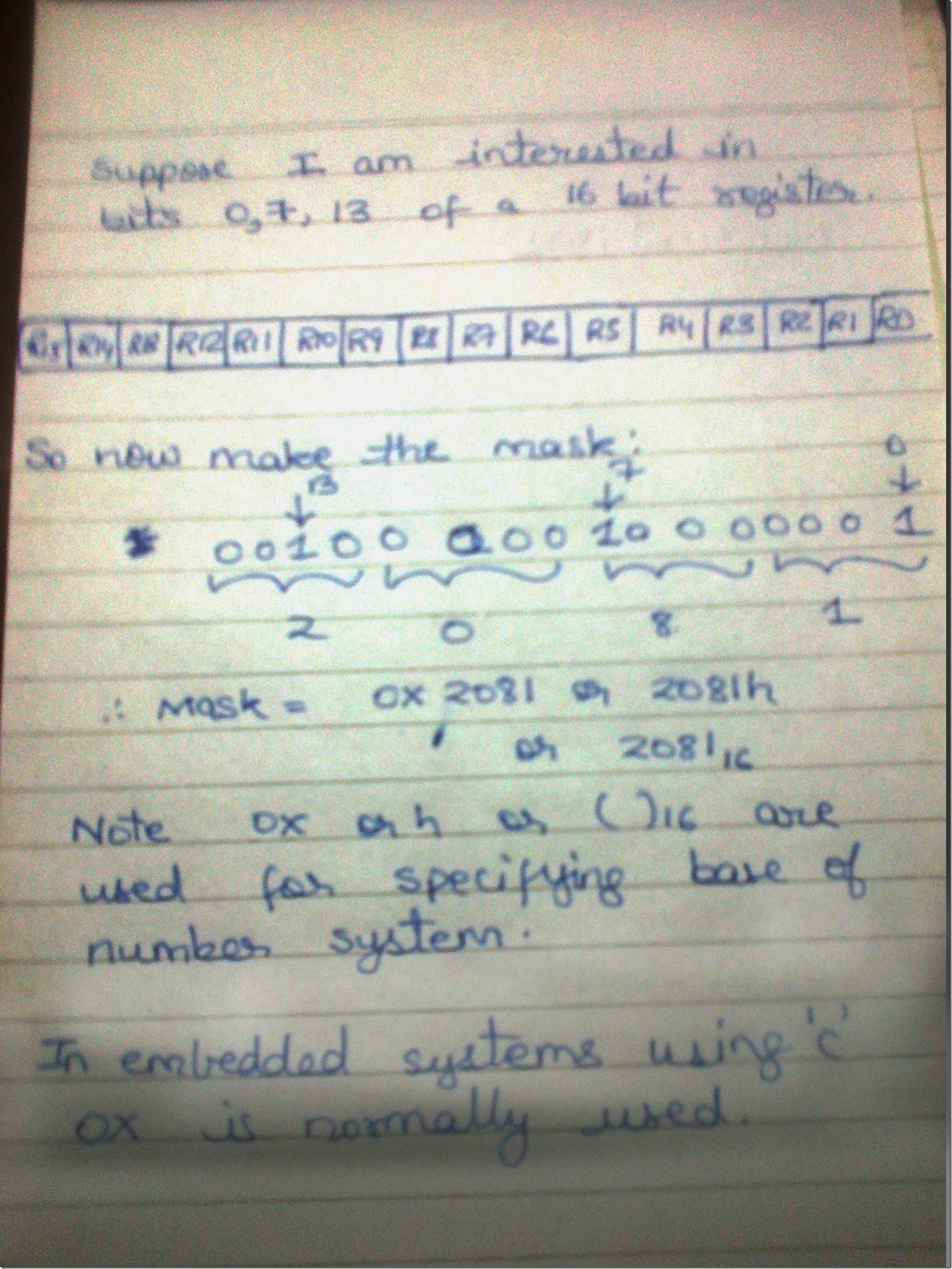
R &= 0x2081 // This is the masking operation in embedded c
R |= 0x2081 // This will set the bits 0,7,13
R ^= 0x2081 // This will toggle bits 0,7,13
So as you can see this example covers masking. Similarly you need to make the bit patterns for bit set, reset and toggle operation.
If you want more examples comment here. Please like the posts if you like what I have written. And do subscribe to get notifications of my new posts.